How to convert user input to uppercase in Javascript
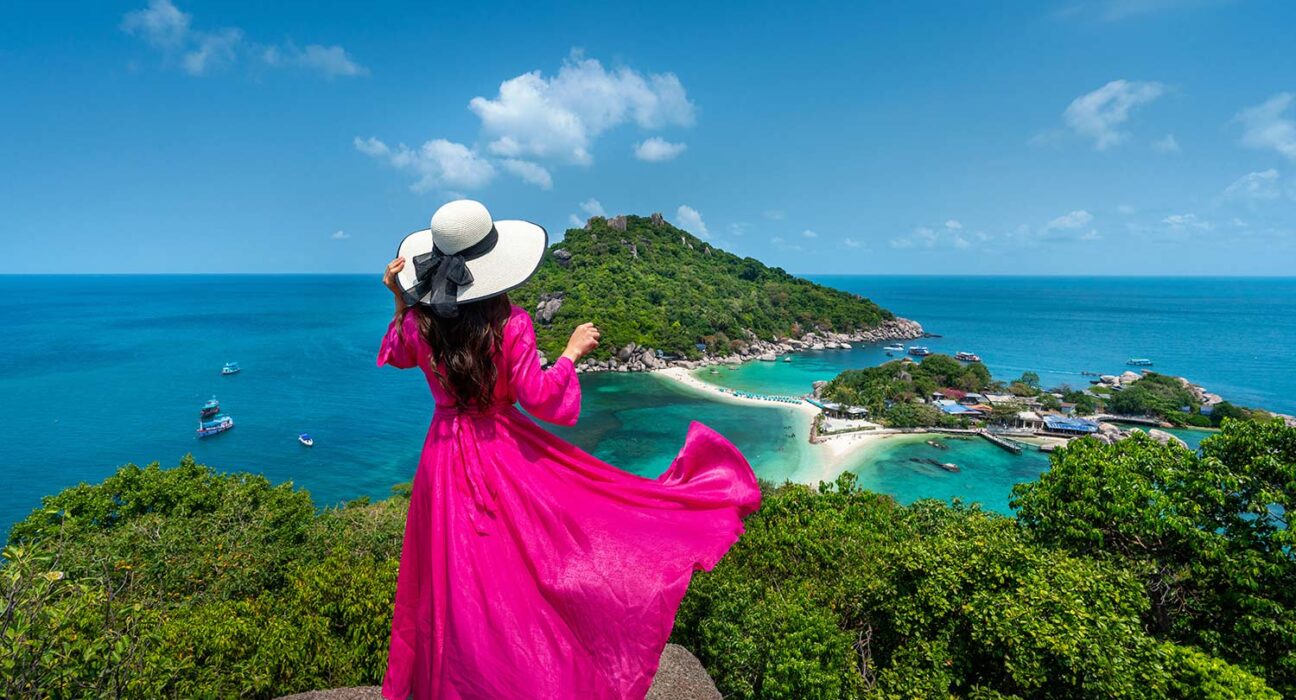
JavaScript provides straightforward methods to manipulate strings, including converting user input to uppercase. This functionality is useful for ensuring consistency in data or formatting text as needed. Let’s explore various ways to convert user input to uppercase in JavaScript.
Using toUpperCase()
Method
1. Using toUpperCase()
for Entire Input
The toUpperCase()
method converts all characters in a string to uppercase:
// Get user input from an input field or prompt
let userInput = 'example input';
// Convert user input to uppercase
let uppercaseInput = userInput.toUpperCase();
console.log(uppercaseInput); // Output: 'EXAMPLE INPUT'
2. Handling User Input Events
When dealing with user input events (like input
or change
), you can convert the input value to uppercase dynamically:
// Assuming there's an input element with the id 'userInput'
const inputElement = document.getElementById('userInput');
inputElement.addEventListener('input', function(event) {
let userInput = event.target.value;
let uppercaseInput = userInput.toUpperCase();
// Use 'uppercaseInput' as needed (e.g., updating UI, processing data)
});
Using CSS for Visual Representation
For visual representation without altering the actual input value, you can use CSS to display the input text as uppercase:
#userInput {
text-transform: uppercase;
}
This approach applies the CSS text-transform
property to change the visual representation of the text to uppercase without modifying the actual input value.
Conclusion
Converting user input to uppercase in JavaScript is achievable using the toUpperCase()
method, which directly transforms all characters to uppercase. Additionally, using event listeners, you can dynamically convert user input as it’s entered into input fields. Alternatively, CSS offers a way to visually represent text in uppercase without altering the input’s actual value.
Integrate these techniques into your web applications to ensure consistency in text formatting or data manipulation based on user input.
Experiment with these methods and determine the most suitable approach for your specific use case to enhance the user experience or ensure standardized data formatting.
Happy coding with string manipulation in JavaScript! 🚀✨