How to align button in center in HTML with CSS
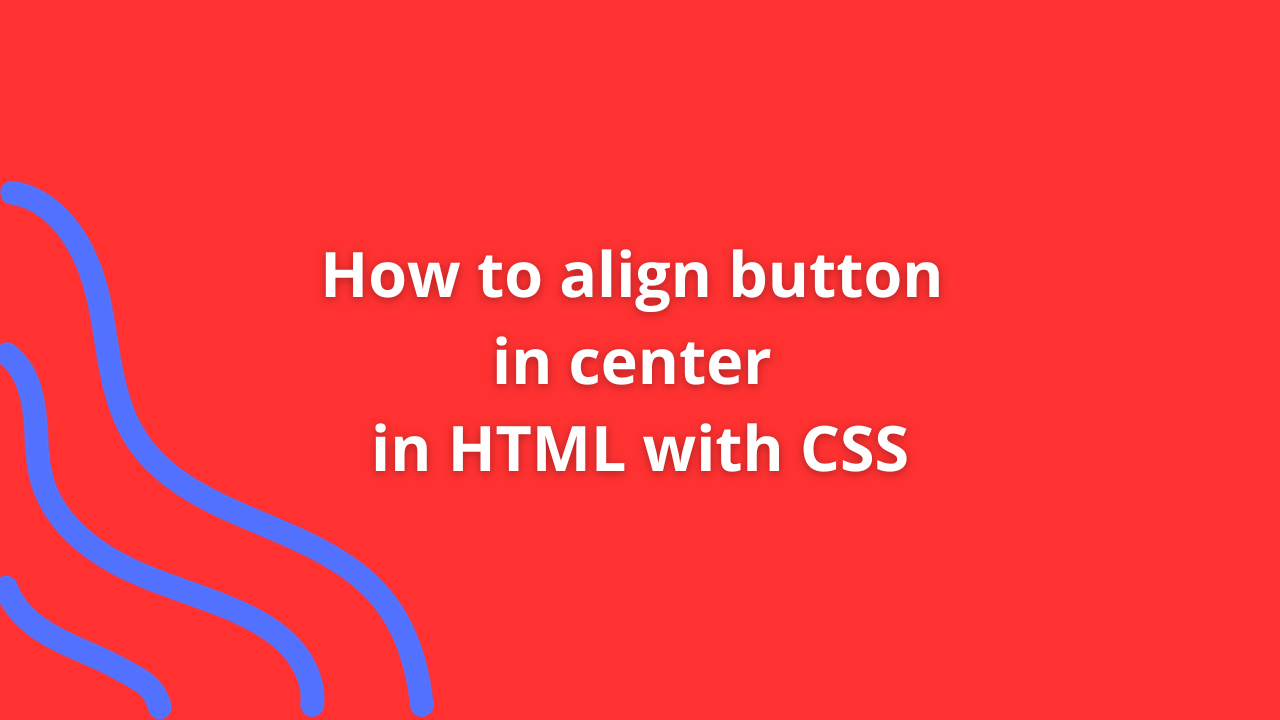
In HTML, buttons are commonly used for various purposes, such as triggering actions, submitting forms, and navigating to different pages. However, aligning a button in the center of a webpage or container can be tricky. In this article, we will explore different methods to align a button in the center of a webpage using CSS.
Method 1: Using text-align property
One of the easiest ways to align a button in the center of a webpage is by using the text-align property in CSS. This property sets the horizontal alignment of an element’s text content. To use this method, you can create a container element, such as a div, and add the button inside it. Then, you can apply the text-align property to the container element and set its value to “center”.
Here is an example code snippet:
<div class="button-container">
<button>Click me</button>
</div>
.button-container {
text-align: center;
}
This method works well if you only have one button to align in the center.
Method 2: Using flexbox
Flexbox is a layout model in CSS that allows you to align and distribute elements in a container. To use flexbox to center a button, you can create a container element, such as a div, and set its display property to “flex”. Then, you can use the justify-content and align-items properties to center the button horizontally and vertically.
Here is an example code snippet:
<div class="button-container">
<button>Click me</button>
</div>
.button-container {
display: flex;
justify-content: center;
align-items: center;
}
This method works well if you have multiple buttons or other elements to align in the center.
Method 3: Using absolute positioning
Absolute positioning allows you to position an element relative to its nearest positioned ancestor. To center a button using absolute positioning, you can create a container element and set its position property to “relative”. Then, you can set the position property of the button to “absolute” and set its top, right, bottom, and left properties to “0”. Finally, you can set the margin property of the button to “auto” to center it horizontally.
Here is an example code snippet:
<div class="button-container">
<button>Click me</button>
</div>
.button-container {
position: relative;
height: 100%; /* set the height of the container to 100% */
}
button {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
margin: auto;
}
This method works well if you want to center the button in a specific position on the webpage.
In conclusion, there are several ways to align a button in the center of a webpage using CSS. You can use the text-align property, flexbox, or absolute positioning depending on your requirements. Experiment with these methods and choose the one that works best for your design.