How to format date in Angular 12
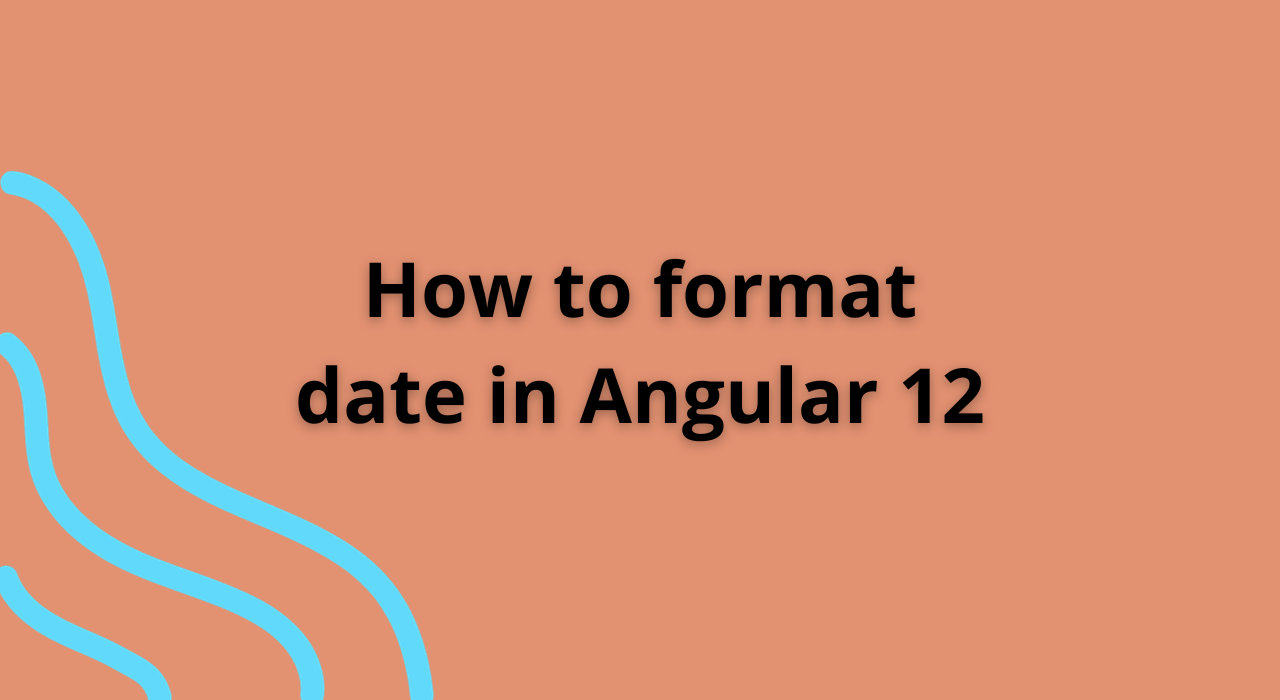
In Angular 12 applications, formatting dates is a common requirement for presenting dates in various formats within the user interface. Angular provides a powerful tool called DatePipe
that facilitates the transformation of date objects into different formats. Let’s explore how to format dates effectively in Angular 12.
Using DatePipe for Date Formatting
1. Import DatePipe
Import DatePipe
from @angular/common
into your component to utilize its functionalities:
import { DatePipe } from '@angular/common';
2. Inject DatePipe into Component
Inject DatePipe
into your component’s constructor:
constructor(private datePipe: DatePipe) { }
3. Format Date
Utilize DatePipe
within your component to format date objects:
// Example usage to format a date
let currentDate = new Date();
let formattedDate = this.datePipe.transform(currentDate, 'dd/MM/yyyy');
console.log(formattedDate); // Output: Formatted date string
4. Applying Custom Formats
Use custom date format strings to achieve specific date representations:
'dd/MM/yyyy'
: Day, month, and year (e.g., 24/11/2023)'EEEE, MMMM d, y'
: Full day name, month name, day of the month, and year (e.g., Thursday, November 24, 2023)
Considerations for Date Formatting
- Locale Settings: Consider setting locale for date formatting based on user preferences or application requirements.
- Time Zone Handling: Handle time zones appropriately when working with dates and times.
- Custom Formatting: Utilize custom format strings to achieve desired date representations.
Conclusion
Angular 12’s DatePipe
provides a robust mechanism for formatting dates, allowing developers to transform date objects into various formats suitable for display within the application’s user interface. By leveraging the functionalities offered by DatePipe
, developers can present dates in a user-friendly and easily interpretable manner.
Incorporate these steps and considerations into your Angular 12 projects to effectively format dates and enhance the presentation of date-related information, catering to diverse user preferences and application requirements. The flexibility offered by DatePipe
empowers developers to display dates in formats that best suit their applications.