How to disable a button if input field is empty in JavaScript
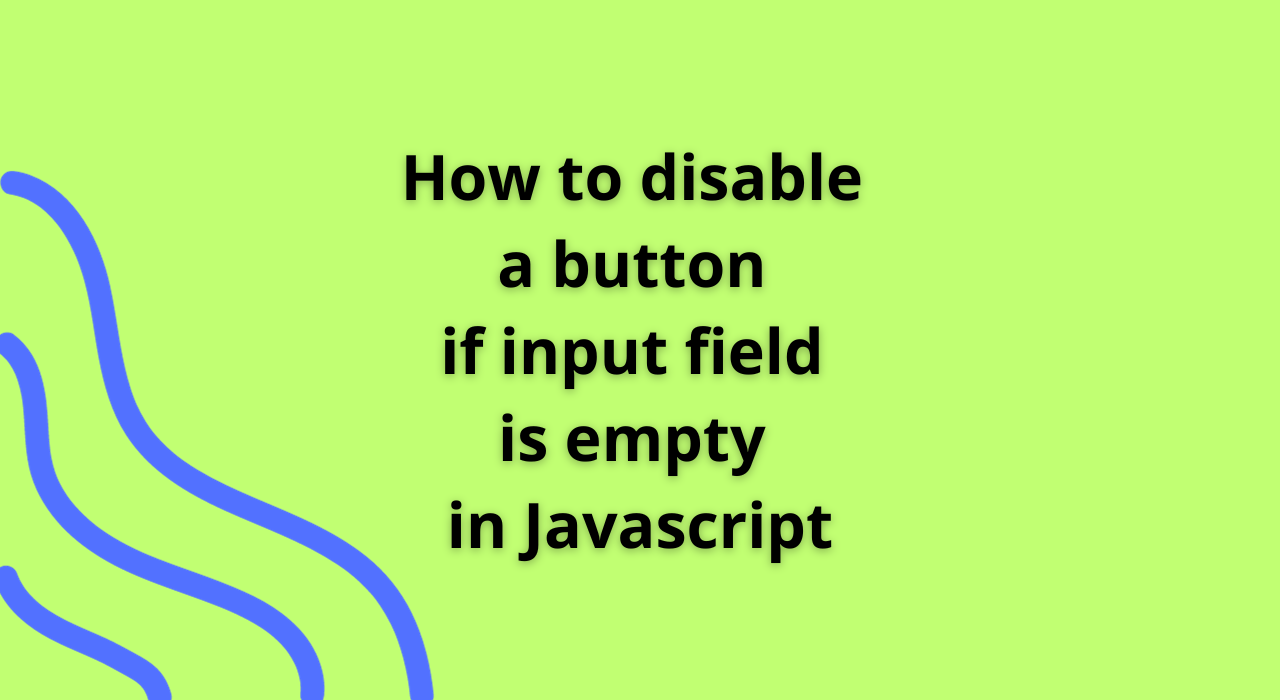
Disabling a button until certain conditions, such as filling out an input field, are met enhances user experience and ensures data integrity. JavaScript provides a straightforward way to disable a button dynamically based on an input field’s content. Let’s explore how to accomplish this.
HTML Structure
Consider an example where you have an input field and a button in your HTML:
<input type="text" id="myInput" placeholder="Type something...">
<button id="myButton" disabled>Submit</button>
In this case, the button starts as disabled (disabled
attribute) by default.
JavaScript Implementation
You can utilize JavaScript to monitor changes in the input field and enable/disable the button accordingly.
const inputField = document.getElementById('myInput');
const submitButton = document.getElementById('myButton');
inputField.addEventListener('input', function() {
// Check if the input field is empty
if (inputField.value.trim() !== '') {
// Enable the button if the input field has content
submitButton.removeAttribute('disabled');
} else {
// Disable the button if the input field is empty
submitButton.setAttribute('disabled', true);
}
});
Explanation
- The
addEventListener
method is used to listen for input changes in the specified input field (myInput
). - When the input event occurs (typing, pasting, etc.), the associated function checks if the input field is empty.
- If the input field contains content (after trimming any leading/trailing spaces), the button is enabled by removing the
disabled
attribute. - If the input field is empty, the button remains disabled by setting the
disabled
attribute.
Test and Refinement
Test this functionality in your browser to ensure the button’s state changes as expected based on the input field’s content. Make adjustments as needed to fit your specific use case or UI design.
Conclusion
Disabling a button until an input field is filled provides users with clear guidance on expected actions and prevents incomplete data submissions. JavaScript empowers developers to create dynamic user interfaces by controlling button states based on user input.
Implement this functionality in your web forms to enhance user experience and maintain data integrity, ensuring users only interact with buttons when necessary information is provided.
Happy coding! 🚀✨