How to set height auto in React Native
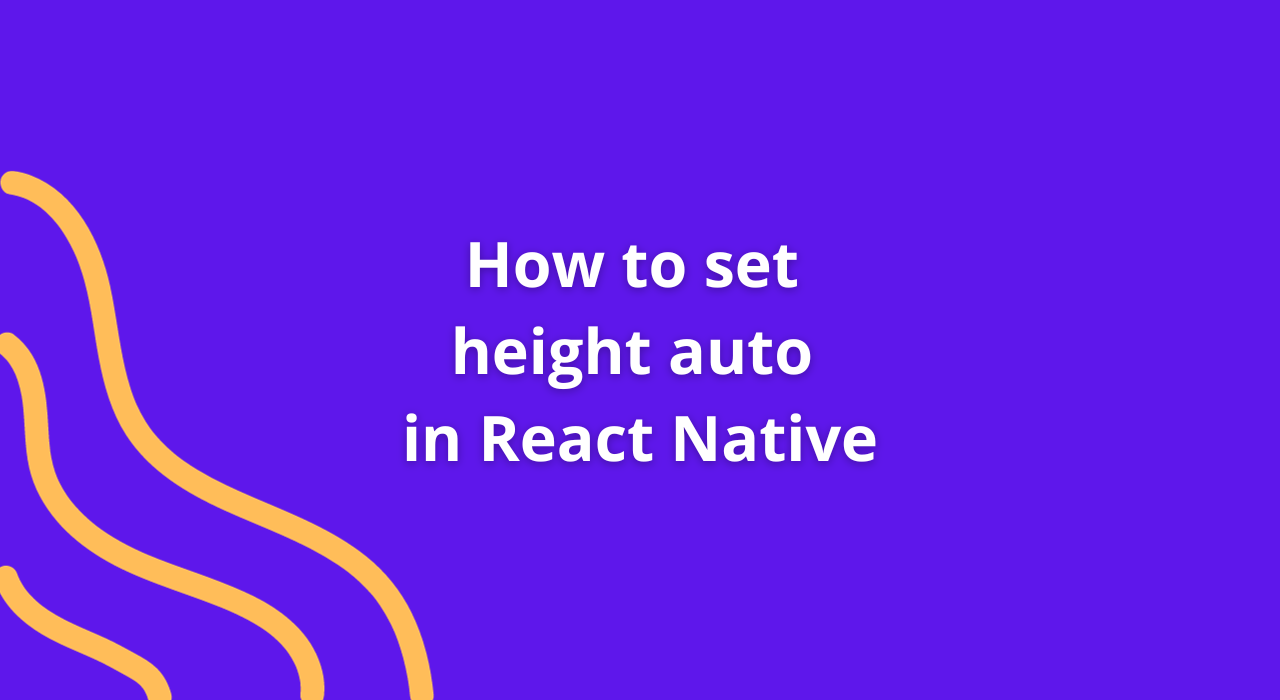
In React Native, managing layout designs and ensuring responsive components often involves handling height adjustments dynamically. Achieving an “auto” height setting is a common requirement for various UI elements. Let’s explore the steps to set height to “auto” in React Native, facilitating flexible and adaptive layouts.
Understanding “Auto” Height in React Native
Setting height to “auto” in React Native allows components to adjust their height dynamically based on their content, ensuring a more responsive and adaptive UI.
Implementing “Auto” Height in React Native
1. Using Flexbox
Utilize Flexbox, a powerful layout system in React Native, to achieve “auto” height for components.
import React from 'react';
import { View, Text, ScrollView } from 'react-native';
const AutoHeightComponent = () => {
return (
<ScrollView contentContainerStyle={{ flexGrow: 1 }}>
<View style={{ flex: 1 }}>
<Text>
Your dynamic content here... Lorem ipsum dolor sit amet, consectetur adipiscing elit.
</Text>
</View>
</ScrollView>
);
};
export default AutoHeightComponent;
2. Using Flex Grow
Apply flexGrow
to enable components to expand vertically based on their content.
import React from 'react';
import { View, Text } from 'react-native';
const AutoHeightComponent = () => {
return (
<View style={{ flex: 1 }}>
<Text style={{ flex: 1 }}>
Your dynamic content here... Lorem ipsum dolor sit amet, consectetur adipiscing elit.
</Text>
</View>
);
};
export default AutoHeightComponent;
3. Setting Height Dynamically
Determine content dynamically or based on user input to achieve variable heights.
import React, { useState } from 'react';
import { View, Text } from 'react-native';
const DynamicHeightComponent = () => {
const [content, setContent] = useState('Your dynamic content here...');
return (
<View style={{ flex: 1 }}>
<Text>
{content}
</Text>
</View>
);
};
export default DynamicHeightComponent;
Considerations and Enhancements
- ScrollView vs. View: Use ScrollView for scrollable content and View for non-scrollable content.
- Layout Structure: Adjust layout structure and style properties to accommodate dynamic content and achieve the desired “auto” height effect.
- Handling Overflow: Ensure content doesn’t overflow beyond the designated container by setting appropriate styles.
Conclusion
Setting height to “auto” in React Native enables components to adjust their height dynamically, catering to varying content lengths and creating more responsive UIs. By leveraging Flexbox and appropriate style properties, developers can achieve flexible layouts that adapt to content changes seamlessly.
Happy Coding !