How to display JSON data in table format using Javascript
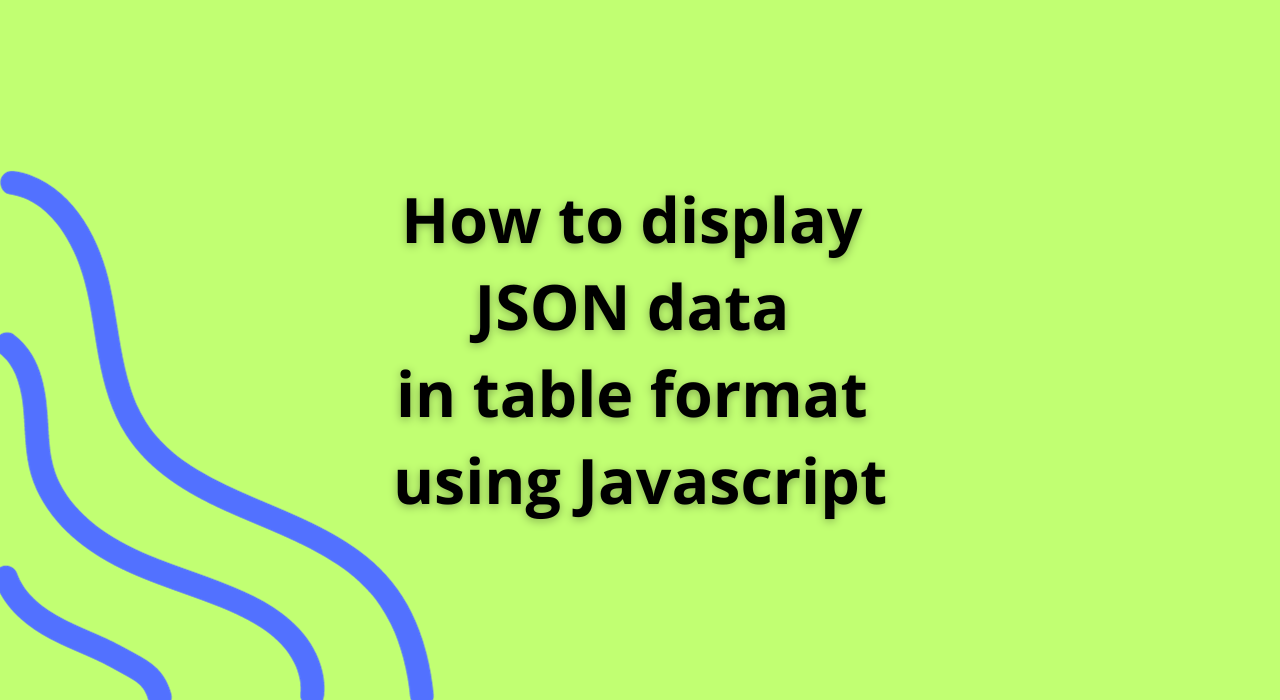
Presenting JSON data in a table format on a webpage can significantly enhance data visualization and readability. JavaScript provides a straightforward method to dynamically generate HTML tables from JSON data. Let’s explore the steps to achieve this effect.
Understanding JSON Data
JSON (JavaScript Object Notation) is a popular data format used for structuring and transmitting data. It consists of key-value pairs, arrays, and nested objects, making it versatile for representing structured data.
HTML Structure
Start with an empty table element in your HTML file:
<table id="data-table"></table>
JavaScript Logic
1. Prepare JSON Data
Create a sample JSON dataset to be displayed in the table:
const jsonData = [
{ id: 1, name: 'John Doe', age: 30, email: 'john@example.com' },
{ id: 2, name: 'Alice Smith', age: 28, email: 'alice@example.com' },
// Add more JSON objects as needed
];
2. Generate Table from JSON Data
Write JavaScript code to dynamically generate a table from the JSON dataset:
function generateTable() {
const table = document.getElementById('data-table');
const headers = Object.keys(jsonData[0]);
// Create table header
let headerRow = table.insertRow();
headers.forEach(headerText => {
let headerCell = headerRow.insertCell();
headerCell.textContent = headerText.toUpperCase();
});
// Create table rows and cells
jsonData.forEach(obj => {
let row = table.insertRow();
headers.forEach(header => {
let cell = row.insertCell();
cell.textContent = obj[header];
});
});
}
Object.keys(jsonData[0])
retrieves the keys (column headers) from the first object in the JSON array.insertRow()
andinsertCell()
are DOM methods to create table rows and cells dynamically.forEach
loops populate header cells with column names and table cells with corresponding JSON values.
3. Call the Function
Invoke the generateTable
function to generate the table from the JSON data:
generateTable();
Testing the Functionality
Include the provided HTML structure and JavaScript logic within your HTML file or separate files and ensure that the jsonData
variable contains valid JSON data.
Conclusion
Displaying JSON data in a table format using JavaScript offers a convenient way to present structured information on webpages. This approach dynamically generates HTML tables, allowing for easy visualization and comprehension of JSON datasets.