How to modify and update data table row in Angular 6
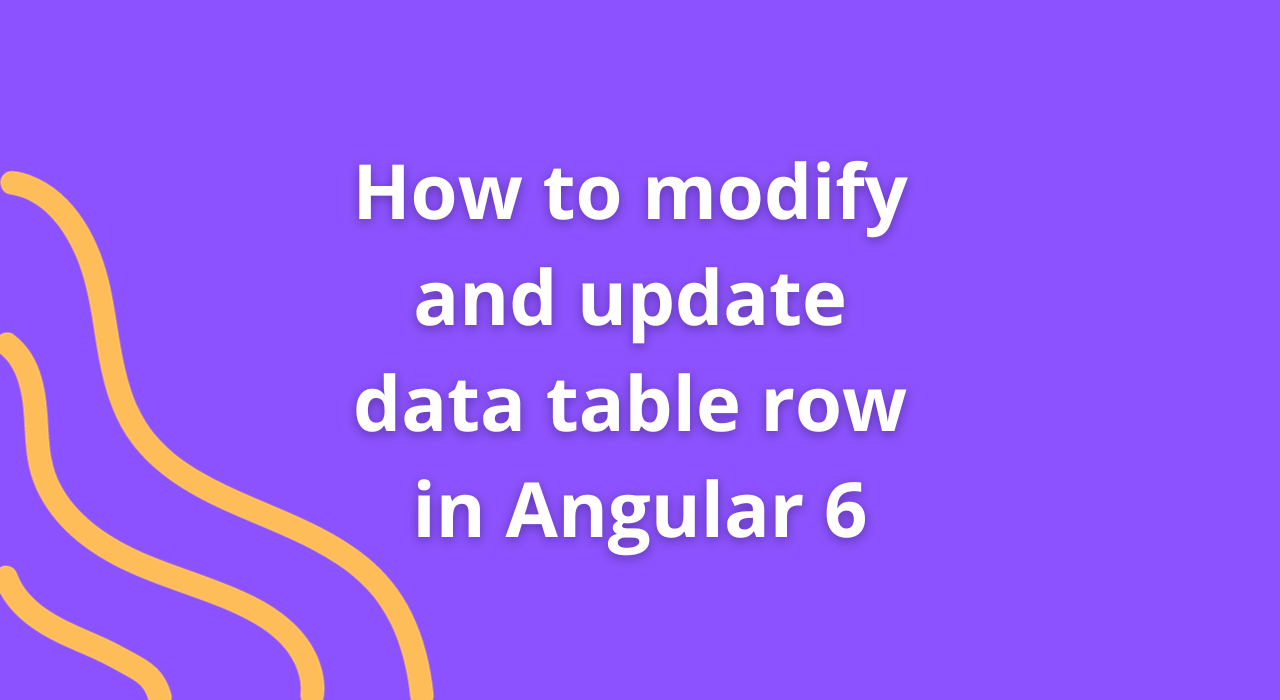
In Angular 6 applications, modifying and updating data table rows involves handling the data model, implementing user interactions, and updating the view dynamically. Let’s dive into the steps required to achieve this functionality within your Angular 6 project.
Setting Up the Angular Project
Ensure you have an Angular 6 project set up and a data table component displaying the relevant data.
Implementing Data Table Row Modification
1. Data Model Setup
Ensure you have a data model or service handling the data displayed in the table. This could be an array of objects or data fetched from an API.
2. Adding Edit Functionality
Implement an edit button or action that triggers the modification mode for a specific row.
3. Modifying Row Data
Upon triggering the edit mode, allow users to modify the data within the row. This could involve form inputs or a modal dialog to edit row-specific information.
4. Updating Data
Implement functionality to update the data within the row. Upon user confirmation or submission, update the corresponding data model or service with the modified values.
5. Reflecting Changes in the View
Ensure the changes made to the data model reflect immediately in the view. Angular’s data binding will update the table rows automatically when the underlying data changes.
Code Example (Partial Representation)
Here’s an example showcasing an approach to modifying and updating data table rows in Angular 6:
// Sample Component Code
export class DataTableComponent {
data: any[] = []; // Sample data array
editMode: boolean = false;
editedRowIndex: number = -1;
// Function to toggle edit mode for a row
toggleEditMode(index: number) {
this.editMode = true;
this.editedRowIndex = index;
}
// Function to save changes and update row data
saveChanges() {
// Update data based on the editedRowIndex
// Example: this.data[this.editedRowIndex] = updatedData;
this.editMode = false;
this.editedRowIndex = -1;
}
}
Conclusion
Modifying and updating data table rows in Angular 6 involves managing the data model, implementing edit functionality, updating the data, and reflecting the changes in the view. This ensures a seamless user experience, allowing users to modify specific rows within the table dynamically.
By following these steps and incorporating appropriate data binding mechanisms, Angular developers can create interactive and user-friendly data tables that facilitate efficient data modification and update operations within their applications.