How to loop through images in Javascript
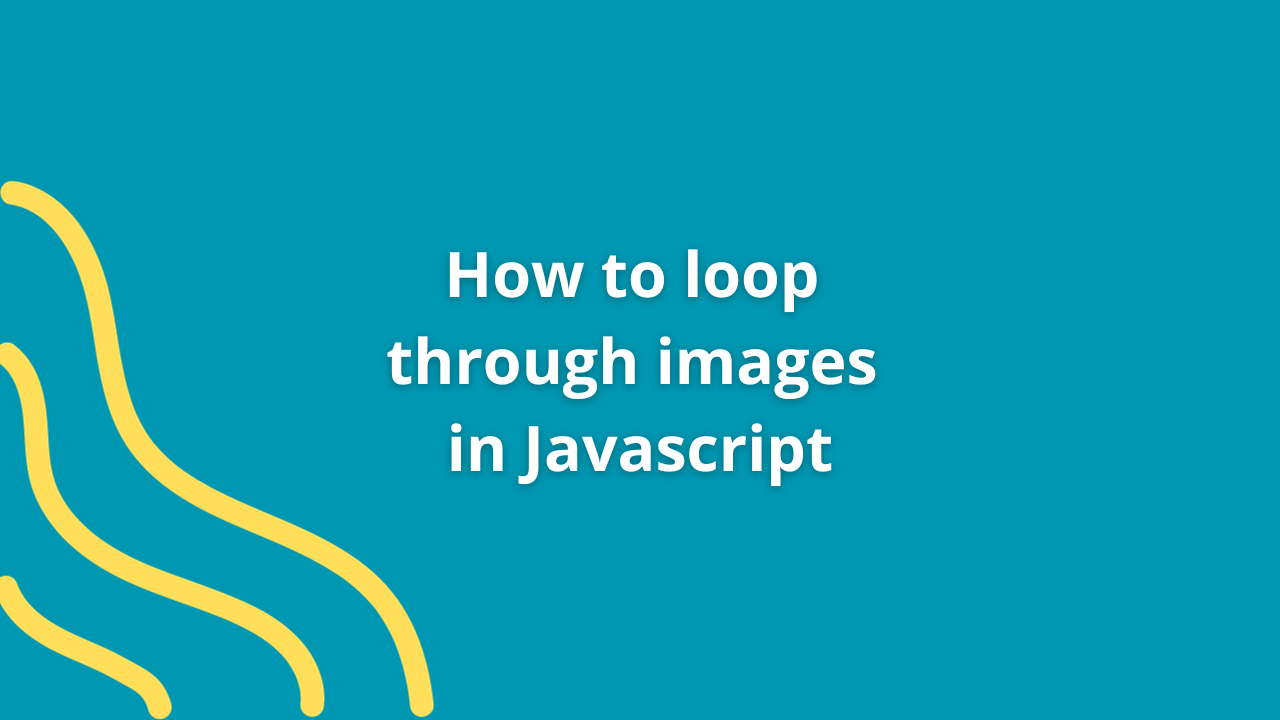
Looping through images in JavaScript enables developers to create engaging visual experiences, such as slideshows, galleries, and interactive image displays. JavaScript’s versatility allows for seamless iteration through image arrays, facilitating dynamic image handling within web applications. Let’s delve into the step-by-step process of looping through images using JavaScript.
Looping Through Images: Step-by-Step Guide
1. Create an Array of Image URLs
Begin by defining an array containing URLs or paths to the images you want to loop through. This array will serve as the source for the images you’ll be displaying.
const imageUrls = [
'path/to/image1.jpg',
'path/to/image2.jpg',
'path/to/image3.jpg',
// Add more image URLs as needed
];
2. Display Images in a Container
Create an HTML container element where the images will be displayed. This could be a div or any other suitable HTML element.
<div id="image-container"></div>
3. Loop Through Image URLs and Display Images
Use JavaScript to loop through the array of image URLs and dynamically create image elements to display the images.
const imageContainer = document.getElementById('image-container');
imageUrls.forEach(url => {
const img = new Image(); // Create a new image element
img.src = url; // Set the image source to the URL
img.alt = 'Image'; // Set alternative text for accessibility
imageContainer.appendChild(img); // Append the image to the container
});
Adding Looping Functionality
1. Set a Timer for Image Switching
To create a slideshow effect, utilize setInterval()
or setTimeout()
to switch between images at defined intervals.
let currentIndex = 0;
function switchImage() {
const imgElements = document.querySelectorAll('#image-container img');
imgElements[currentIndex].style.display = 'none'; // Hide current image
currentIndex = (currentIndex + 1) % imageUrls.length; // Update index for the next image
imgElements[currentIndex].style.display = 'block'; // Display the next image
}
// Set an interval to switch images every 3 seconds (adjust the time as needed)
setInterval(switchImage, 3000);
2. Handling Image Wrap-Around
Ensure the images loop back to the beginning after reaching the end of the array by resetting the index.
currentIndex = (currentIndex + 1) % imageUrls.length;
Advanced Techniques and Considerations
- Adding Transitions: Implement CSS transitions or JavaScript animation libraries for smooth image transitions.
- User Controls: Incorporate buttons or controls to allow users to pause, play, or navigate through images manually.
- Optimizing Image Loading: Optimize image loading techniques, such as lazy loading, to enhance performance, especially for larger image sets.
Conclusion
Looping through images in JavaScript empowers developers to create dynamic and engaging visual content within web applications. By leveraging JavaScript’s iteration capabilities and DOM manipulation, developers can craft immersive slideshows, image galleries, and interactive image displays.
You Might Also Like
- Lorem ipsum dolarorit ametion consectetur
- The Blues Kitchen woks Podcast
- Chasing Dreams in Slow Motion
- If you use this site regularly and would like to help keep the site on the Internet,
- Dolarorit ametion consectetur elit.
- Modern Office Must-Have in 2021
- If you are going to use a passage of Lorem
- Lorem ipsum consectetur elit.