How to implement autocomplete search box in React JS
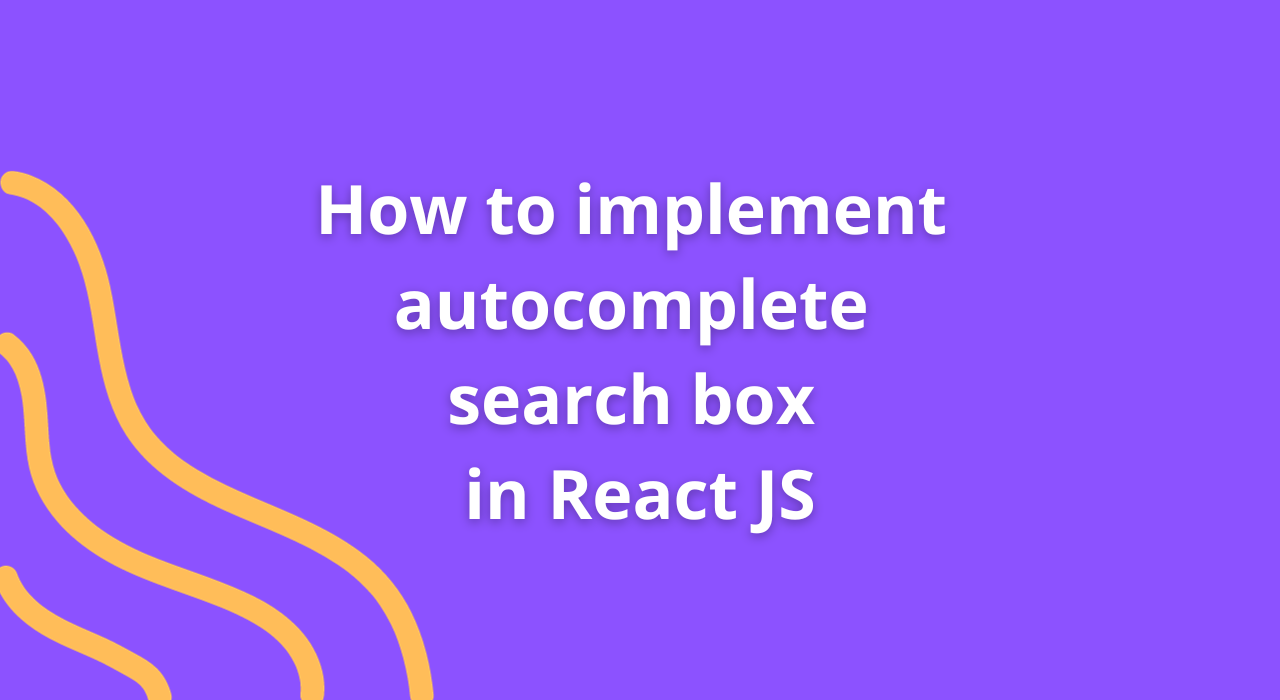
Autocomplete search boxes offer users a seamless and intuitive way to find information efficiently within web applications. Implementing this functionality in React.js enhances user experience by providing real-time suggestions as users type. Let’s delve into the step-by-step process of creating an autocomplete search box in React.js, leveraging its state management and lifecycle methods.
Setting Up the React Application
Start by setting up a React application using Create React App or any preferred method.
npx create-react-app autocomplete-search-app
cd autocomplete-search-app
Implementing the Autocomplete Search Box
1. Create a Search Component
Create a component to handle the autocomplete search functionality.
import React, { useState } from 'react';
const AutocompleteSearch = () => {
const [searchQuery, setSearchQuery] = useState('');
const [suggestions, setSuggestions] = useState([]);
// Function to handle input change
const handleInputChange = (event) => {
const value = event.target.value;
setSearchQuery(value);
// Implement search logic and update suggestions based on 'value'
// Example: Fetch suggestions from an API endpoint or a local data source
};
return (
<div>
<input
type="text"
value={searchQuery}
onChange={handleInputChange}
placeholder="Search..."
/>
{/* Display suggestions */}
<ul>
{suggestions.map((suggestion, index) => (
<li key={index}>{suggestion}</li>
))}
</ul>
</div>
);
};
export default AutocompleteSearch;
2. Fetch Suggestions
Implement the logic to fetch suggestions based on the user’s input (e.g., querying an API or filtering data locally).
3. Update Suggestions
Update the suggestions
state based on the fetched data to display suggestions dynamically as the user types.
Styling and Enhancements
- Styling: Apply CSS styles to enhance the search box and suggestions display for a better user interface.
- Debouncing or Throttling: Implement debouncing or throttling techniques to limit API calls and optimize performance, especially for frequent user input.
Integration into Application
Integrate the AutocompleteSearch
component into your application by importing and rendering it within the desired parent component.
Conclusion
Implementing an autocomplete search box in React.js offers users a user-friendly way to find information efficiently. Leveraging React’s state management and lifecycle methods, developers can create dynamic and responsive autocomplete functionality that enhances the user experience within web applications.