How to fetch data from database in React JS using axios
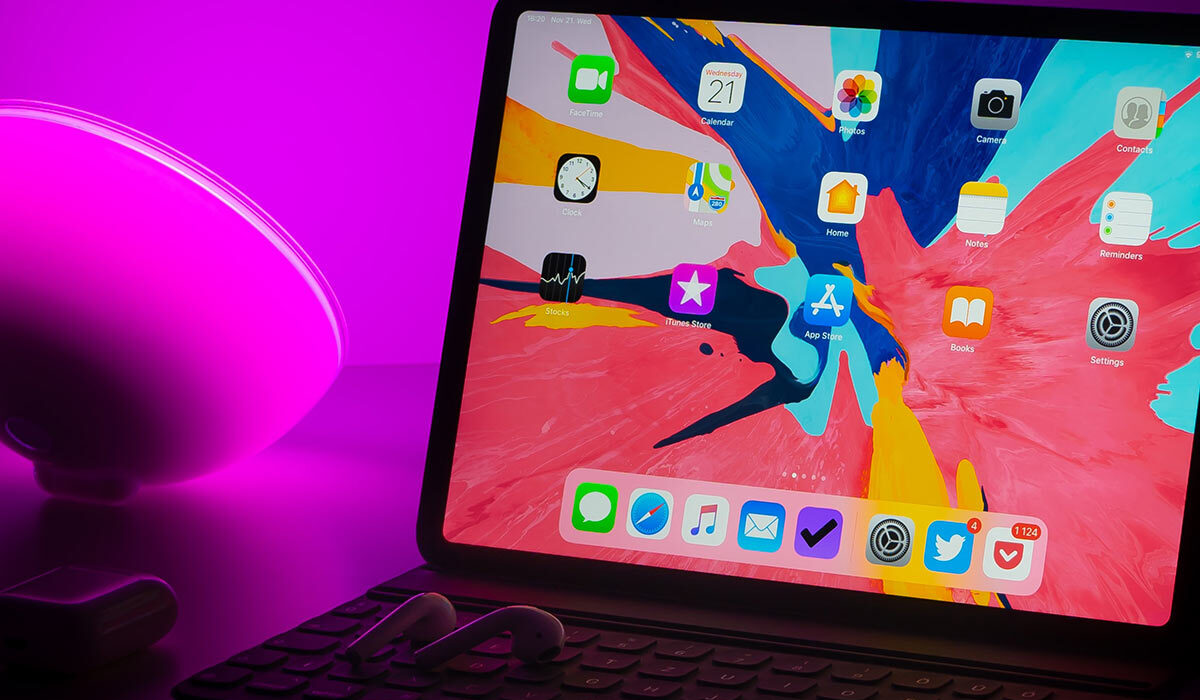
In React.js applications, fetching data from a database or API is a fundamental operation for creating dynamic and interactive user experiences. Leveraging tools like Axios simplifies the process, enabling seamless data retrieval from databases. Let’s explore how to fetch data from a database in React.js using Axios, a popular HTTP client.
Setting Up Axios in React.js
1. Install Axios
Start by installing Axios, a promise-based HTTP client, using npm or yarn within your React.js project.
npm install axios
# or
yarn add axios
2. Import Axios
Import Axios into your React component where you intend to fetch data.
import axios from 'axios';
Fetching Data with Axios in React.js
1. Performing a GET Request
Use Axios to perform a GET request to retrieve data from a database or an API endpoint.
axios.get('https://your-api-endpoint')
.then(response => {
// Handle data fetched successfully
console.log(response.data); // Log fetched data to the console
// Set the fetched data to state or perform other operations
})
.catch(error => {
// Handle errors during data fetching
console.error('Error fetching data:', error);
});
2. Using Async/Await
Alternatively, you can use async/await syntax for cleaner asynchronous code.
async function fetchData() {
try {
const response = await axios.get('https://your-api-endpoint');
console.log(response.data); // Log fetched data to the console
// Set the fetched data to state or perform other operations
} catch (error) {
console.error('Error fetching data:', error);
}
}
3. Integrating with React Component
Integrate data fetching into your React component’s lifecycle methods (e.g., useEffect
for functional components or componentDidMount
for class components) to initiate data retrieval.
Handling Fetched Data in React Components
Once data is fetched using Axios, manage the retrieved data within your React components. You can update state variables, trigger re-renders, or pass the data down as props to child components for rendering.
Conclusion
Axios simplifies the process of fetching data from databases or APIs within React.js applications. By harnessing its capabilities for making HTTP requests, developers can seamlessly integrate data fetching into their components, enabling the creation of dynamic and responsive user interfaces.
You Might Also Like
- Lorem ipsum dolarorit ametion consectetur
- The Blues Kitchen woks Podcast
- Chasing Dreams in Slow Motion
- If you use this site regularly and would like to help keep the site on the Internet,
- Dolarorit ametion consectetur elit.
- Modern Office Must-Have in 2021
- If you are going to use a passage of Lorem
- Lorem ipsum consectetur elit.