How to fetch data from database in Javascript and display in HTML table
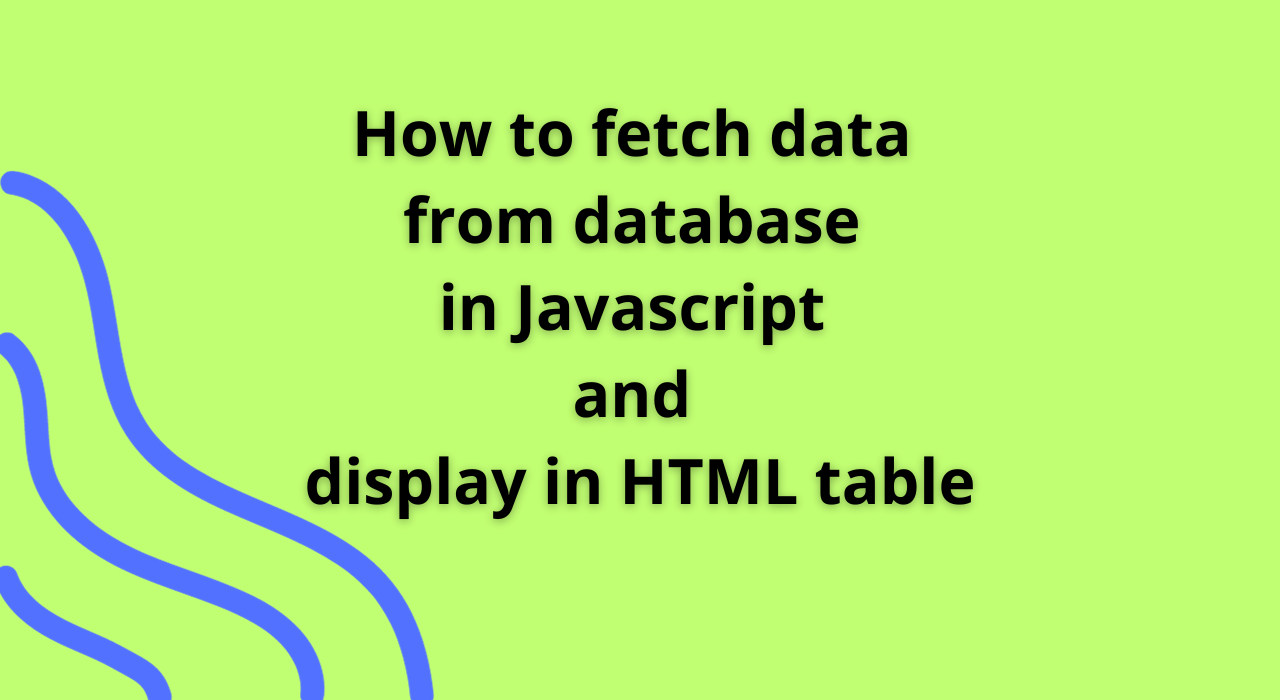
In modern web development, the ability to retrieve data from databases and present it dynamically on web pages is essential. JavaScript, combined with various backend technologies, enables fetching data from databases and seamlessly displaying it in HTML tables. Let’s explore the process of fetching database data using JavaScript and showcasing it in an HTML table.
Setting Up the Environment
Before diving into the process, ensure you have a backend server and a database set up. Common choices for databases include MySQL, PostgreSQL, MongoDB, or SQLite. Establish an API endpoint on the server to fetch data from the database.
Fetching Data Using JavaScript
Using Fetch API
The Fetch API simplifies making HTTP requests, including fetching data from APIs or backend endpoints. Employ the Fetch API to retrieve data from the server.
fetch('https://your-api-endpoint')
.then(response => response.json())
.then(data => {
// Process the retrieved data
displayDataInTable(data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Displaying Data in an HTML Table
Upon successfully fetching data, create a function to display it in an HTML table. This function will manipulate the DOM to generate the table structure and populate it with the fetched data.
function displayDataInTable(data) {
const table = document.createElement('table');
const header = table.createTHead();
const row = header.insertRow();
// Create table headers
for (let key in data[0]) {
const th = document.createElement('th');
th.textContent = key;
row.appendChild(th);
}
// Populate table with data
const tbody = table.createTBody();
data.forEach(item => {
const row = tbody.insertRow();
for (let key in item) {
const cell = row.insertCell();
cell.textContent = item[key];
}
});
// Append table to a container element in the HTML
document.getElementById('table-container').appendChild(table);
}
HTML Structure
Ensure your HTML file includes a container element where the table will be appended:
<div id="table-container"></div>
Security Considerations
When fetching data from a database, security is paramount. Implement proper server-side validation and sanitization to prevent SQL injection attacks or exposure of sensitive information.
Conclusion
Fetching data from databases using JavaScript and displaying it in HTML tables offers dynamic and interactive content for web applications. By leveraging JavaScript’s Fetch API and DOM manipulation, developers can seamlessly retrieve data from servers and present it in an organized, user-friendly manner.