How to create an array of images in Javascript
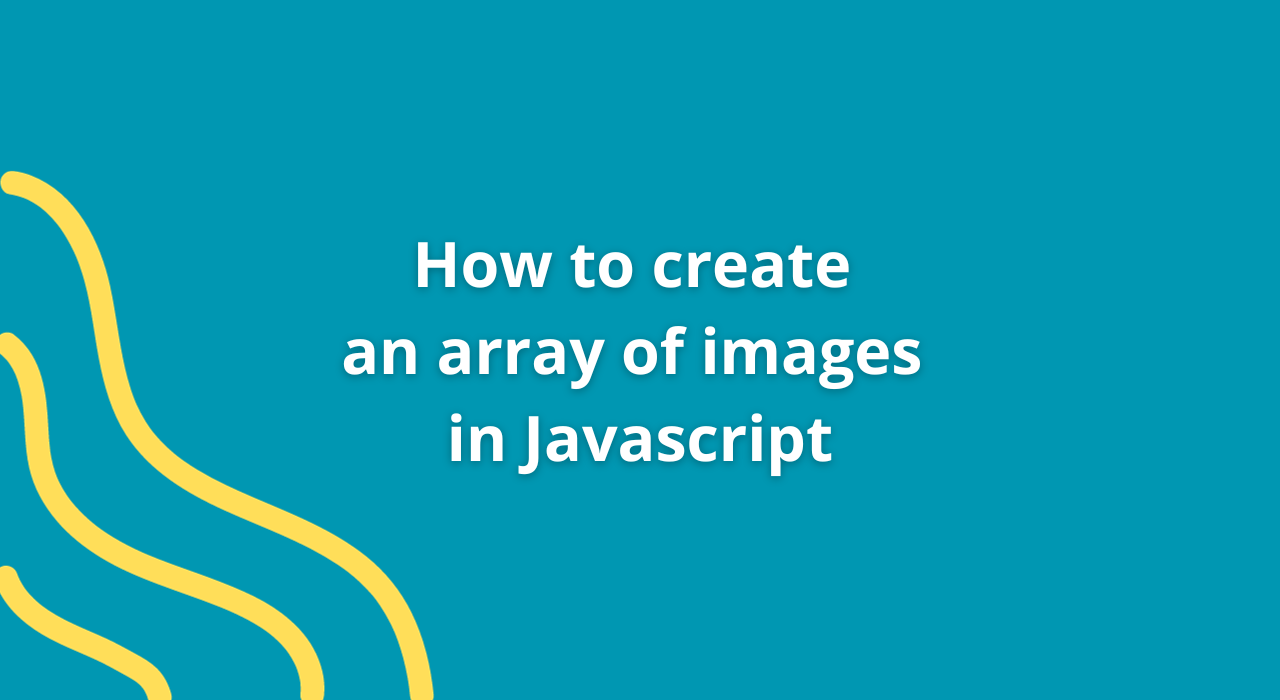
In modern web development, dynamically managing and displaying arrays of images adds a layer of interactivity and visual appeal to web applications. JavaScript offers versatile methods to create arrays containing image elements, enabling developers to manipulate and showcase images seamlessly. Let’s explore the step-by-step process of creating arrays of images in JavaScript.
Creating Image Arrays Using JavaScript
1. Defining Image URLs
Begin by defining URLs or paths to the images you want to include in your array. These can be local paths or URLs pointing to external images.
const imageUrls = [
'path/to/image1.jpg',
'path/to/image2.jpg',
'https://example.com/image3.jpg',
// Add more image URLs as needed
];
2. Creating Image Elements
Iterate through the array of image URLs and create image elements dynamically using JavaScript.
const imageElements = [];
imageUrls.forEach(url => {
const img = new Image(); // Create a new image element
img.src = url; // Set the image source to the URL
imageElements.push(img); // Add the image element to the array
});
3. Manipulating Image Attributes
You can further customize the image elements by setting attributes such as alt
(alternative text), width
, height
, and other properties.
imageElements.forEach(img => {
img.alt = 'Image'; // Set alternative text for accessibility
img.width = 300; // Set a specific width for the images (optional)
img.height = 200; // Set a specific height for the images (optional)
// Add more customizations as required
});
4. Appending Images to HTML
Finally, append the dynamically created image elements to an HTML container, such as a div or section, to display them within the web page.
const imageContainer = document.getElementById('image-container'); // Get the container element
imageElements.forEach(img => {
imageContainer.appendChild(img); // Append each image element to the container
});
Advanced Techniques and Considerations
- Preloading Images: Preloading images using JavaScript can optimize the user experience by ensuring images are loaded and cached before they are displayed.
- Lazy Loading: Implement lazy loading techniques to load images only when they enter the user’s viewport, enhancing page load performance.
- Handling Image Load Errors: Use event listeners (
onload
,onerror
) to handle image load success or failure, providing fallbacks for failed image loads.
Conclusion
JavaScript empowers developers to create dynamic arrays of images, allowing for the manipulation and display of images within web applications. By leveraging JavaScript’s capabilities to create image elements dynamically, developers can create visually engaging experiences for users.
You Might Also Like
- Lorem ipsum dolarorit ametion consectetur
- The Blues Kitchen woks Podcast
- Chasing Dreams in Slow Motion
- If you use this site regularly and would like to help keep the site on the Internet,
- Dolarorit ametion consectetur elit.
- Modern Office Must-Have in 2021
- If you are going to use a passage of Lorem
- Lorem ipsum consectetur elit.