How to convert array of objects to map in Javascript
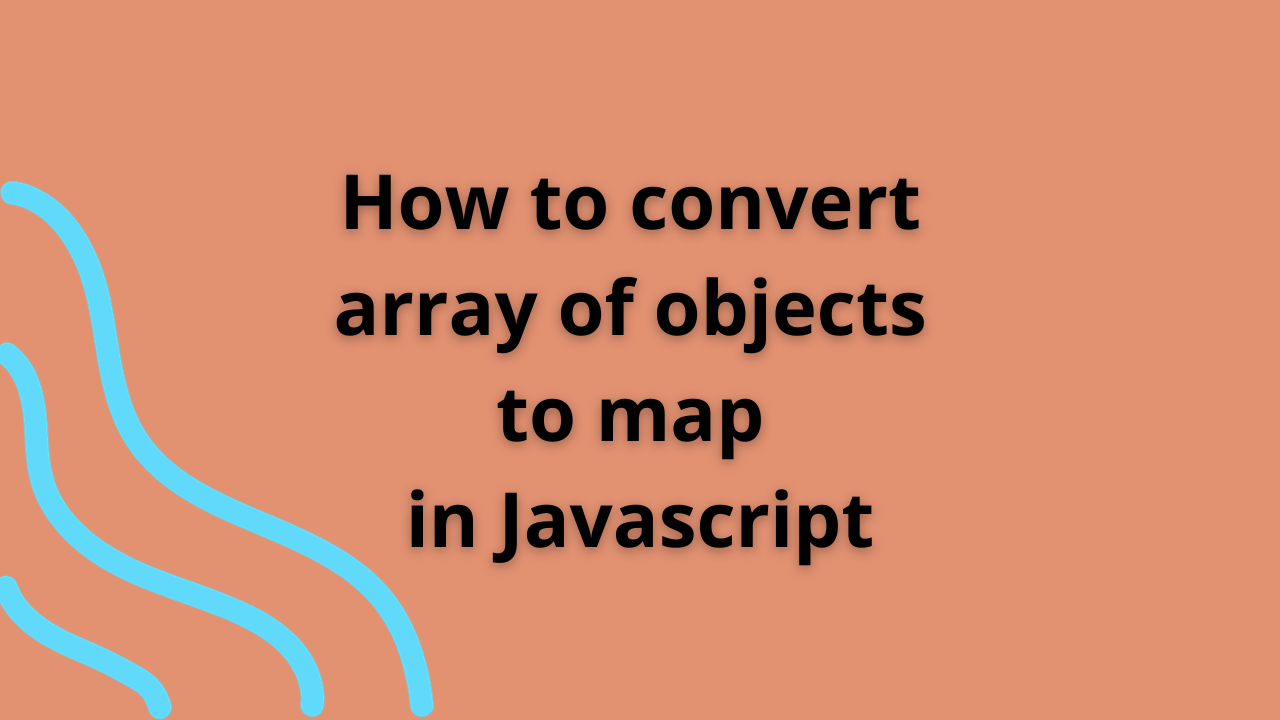
JavaScript offers versatile ways to manipulate data structures. Converting an array of objects to a map can be incredibly useful when organizing data for efficient access or lookup. Let’s delve into the steps to achieve this transformation.
Understanding Arrays and Maps in JavaScript
Arrays
Arrays in JavaScript are ordered collections of values indexed by integers, providing easy access to elements based on their position.
Maps
Maps, introduced in ES6, are collections of key-value pairs where keys can be of any data type, offering efficient data retrieval.
Converting an Array of Objects to a Map
1. Creating an Array of Objects
Consider having an array of objects, each containing a unique identifier:
const arrayOfObjects = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
// Add more objects as needed
];
2. Converting to a Map
Convert the array of objects to a map using the Map
constructor and the forEach
method:
// Convert array of objects to a Map
const mapFromObjects = new Map();
arrayOfObjects.forEach(obj => {
mapFromObjects.set(obj.id, obj);
});
new Map()
creates an empty map..forEach()
iterates through each object in the array.mapFromObjects.set(key, value)
adds each object to the map, using the unique identifier (id
in this case) as the key.
3. Accessing Values in the Map
Once the conversion is complete, access values in the map using their keys:
const result = mapFromObjects.get(2); // Accessing object with key 2
console.log(result); // Output: { id: 2, name: 'Bob' }
mapFromObjects.get(key)
retrieves the value (object) associated with the provided key from the map.
Conclusion
Converting an array of objects to a map in JavaScript facilitates efficient data retrieval based on specific keys. Maps offer flexibility and quicker access compared to traditional arrays, especially when dealing with large datasets.
Utilize this transformation technique to organize and manage data more effectively, catering to scenarios where quick access to object properties based on unique identifiers is essential.
Experiment with different data structures and leverage the power of maps in JavaScript to streamline data management in your applications.