How to Connect Mongoose with NestJS: A Step-by-Step Tutorial
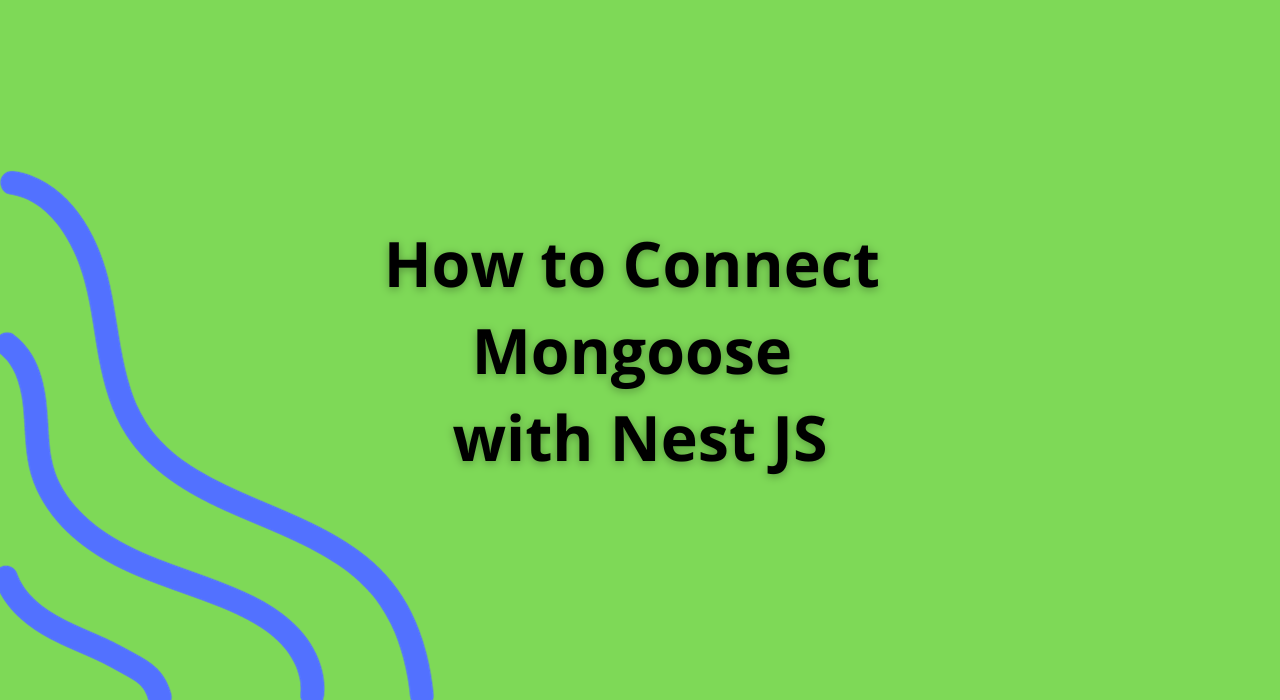
Mongoose, a powerful ODM (Object Data Modeling) library for MongoDB, seamlessly integrates with NestJS to handle database interactions efficiently. Integrating Mongoose with NestJS allows for easy management of MongoDB databases and models within a NestJS application. Let’s explore the steps to connect Mongoose with NestJS.
Prerequisites
Ensure you have Node.js and npm (Node Package Manager) installed on your machine, along with a MongoDB instance accessible to your NestJS application.
Setting Up a NestJS Project
1. Create a New NestJS Project
Generate a new NestJS project using the Nest CLI:
nest new my-nest-app
2. Install Required Dependencies
Navigate to your project directory and install the @nestjs/mongoose
package:
npm install --save @nestjs/mongoose mongoose
This package facilitates seamless integration between NestJS and Mongoose.
Connecting Mongoose to NestJS
1. Configure Database Connection
Open the app.module.ts
file and configure the MongooseModule with your MongoDB connection settings:
import { Module } from '@nestjs/common';
import { MongooseModule } from '@nestjs/mongoose';
@Module({
imports: [
MongooseModule.forRoot('mongodb://localhost:27017/mydatabase'),
// Replace 'mydatabase' with your MongoDB database name
],
})
export class AppModule {}
Adjust the connection URL ('mongodb://localhost:27017/mydatabase'
) to match your MongoDB instance’s URL and database name.
2. Create Mongoose Models
Define Mongoose models representing your MongoDB collections. For example, create a User
model:
import { Schema, Prop, SchemaFactory } from '@nestjs/mongoose';
import { Document } from 'mongoose';
@Schema()
export class User extends Document {
@Prop()
name: string;
@Prop()
email: string;
// Add more properties as needed
}
export const UserSchema = SchemaFactory.createForClass(User);
3. Use Mongoose Models in NestJS Services
Inject the Mongoose models into NestJS services to perform CRUD operations:
import { Injectable } from '@nestjs/common';
import { InjectModel } from '@nestjs/mongoose';
import { Model } from 'mongoose';
import { User, UserDocument } from './user.schema';
@Injectable()
export class UserService {
constructor(@InjectModel(User.name) private readonly userModel: Model<UserDocument>) {}
async findAll(): Promise<User[]> {
return this.userModel.find().exec();
}
// Implement other CRUD operations as needed
}
Conclusion
Connecting Mongoose with NestJS streamlines database interactions and allows for efficient handling of MongoDB data within a NestJS application. This integration facilitates seamless data modeling, CRUD operations, and database management, empowering developers to build robust and scalable applications with ease.