How to make icon clickable in Javascript
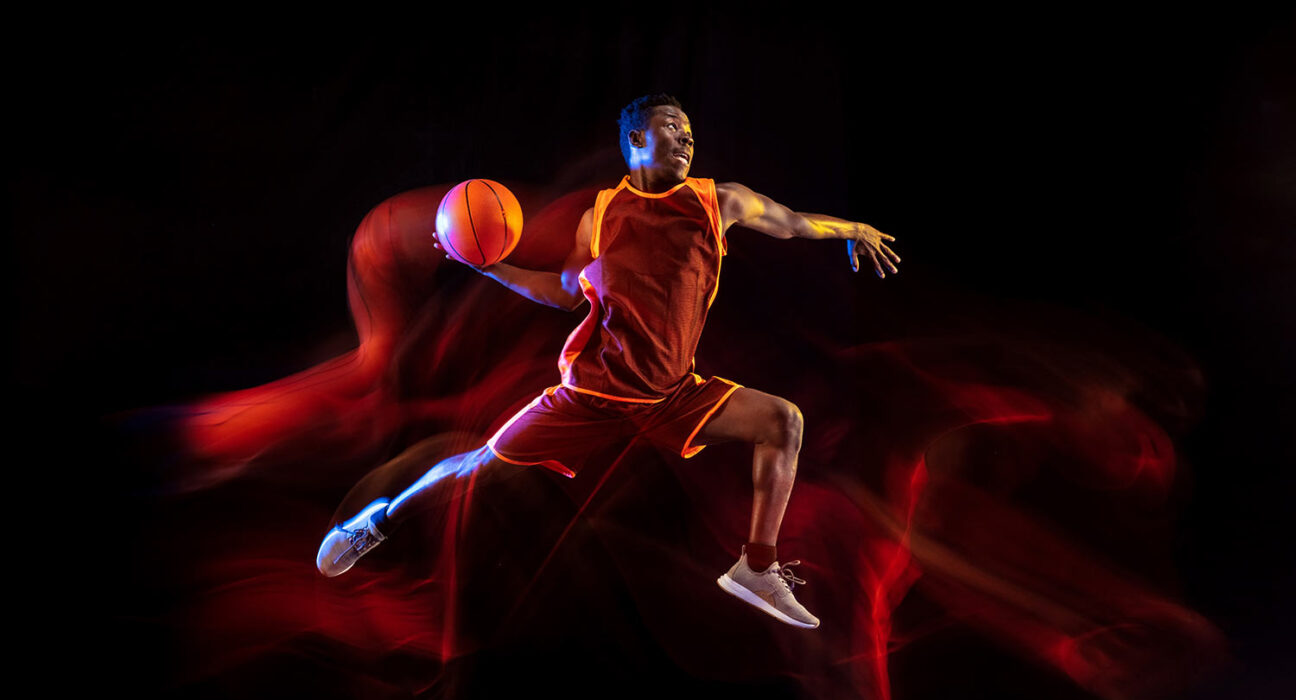
Icons serve as powerful visual elements on web pages, often conveying essential functions or actions to users. Making these icons clickable, triggering specific actions or interactions, can significantly enhance user experience. Let’s delve into the process of making icons clickable using JavaScript, enabling seamless interactivity within web applications.
The Basics of Clickable Icons
1. Selecting the Icon
To make an icon clickable, first, identify the HTML element representing the icon. This could be an <img>
tag, an SVG icon, or an icon rendered using an HTML element with a specific class or ID.
2. Adding Event Listeners
JavaScript allows interaction with HTML elements through event listeners. Attach a click event listener to the selected icon element to detect when it’s clicked by the user.
const icon = document.getElementById('myIcon'); // Replace 'myIcon' with your icon's ID or select it using other methods
icon.addEventListener('click', function() {
// Perform actions upon clicking the icon
// For example, trigger a function or navigate to another page
handleClick();
});
3. Defining Actions
Inside the event listener, define the actions to be executed when the icon is clicked. These actions could range from simple UI interactions to more complex functionalities, such as opening a modal, toggling a menu, submitting a form, or navigating to another page.
function handleClick() {
// Example action: Display an alert upon clicking the icon
alert('Icon clicked! Perform desired action here.');
// Other actions can be placed here based on your application's requirements
}
Enhancing Clickable Icons
Styling for Interaction
Consider adding visual cues to indicate the icon’s clickability, such as changing its appearance when hovered over by the cursor. This can be achieved using CSS, applying styles to the icon element based on different states (e.g., :hover
).
#myIcon {
cursor: pointer; /* Change cursor on hover to indicate clickability */
}
#myIcon:hover {
opacity: 0.8; /* Example: Reduce opacity when hovered */
/* Add additional styles for hover effects */
}
Best Practices and Considerations
- Accessibility: Ensure clickable icons are accessible to all users, including those using assistive technologies. Use appropriate HTML semantics and provide alternative text or labels for icons.
- Consistency: Maintain consistency in icon behavior throughout the application to provide a seamless user experience.
- Testing: Thoroughly test clickable icons across various browsers and devices to ensure they function as intended.
Conclusion
Integrating clickable icons using JavaScript adds interactivity and functionality to web applications, enabling users to engage with specific features or perform desired actions. By leveraging event listeners and defining actions, developers can create intuitive and interactive experiences, enhancing usability and user engagement.
Mastering the art of making icons clickable with JavaScript opens doors to creating more dynamic and user-friendly web interfaces, contributing to an enriched browsing experience for users interacting with your application.
You Might Also Like
- Lorem ipsum dolarorit ametion consectetur
- The Blues Kitchen woks Podcast
- Chasing Dreams in Slow Motion
- If you use this site regularly and would like to help keep the site on the Internet,
- Dolarorit ametion consectetur elit.
- Modern Office Must-Have in 2021
- If you are going to use a passage of Lorem
- Lorem ipsum consectetur elit.