How to create database in MongoDB using Node JS
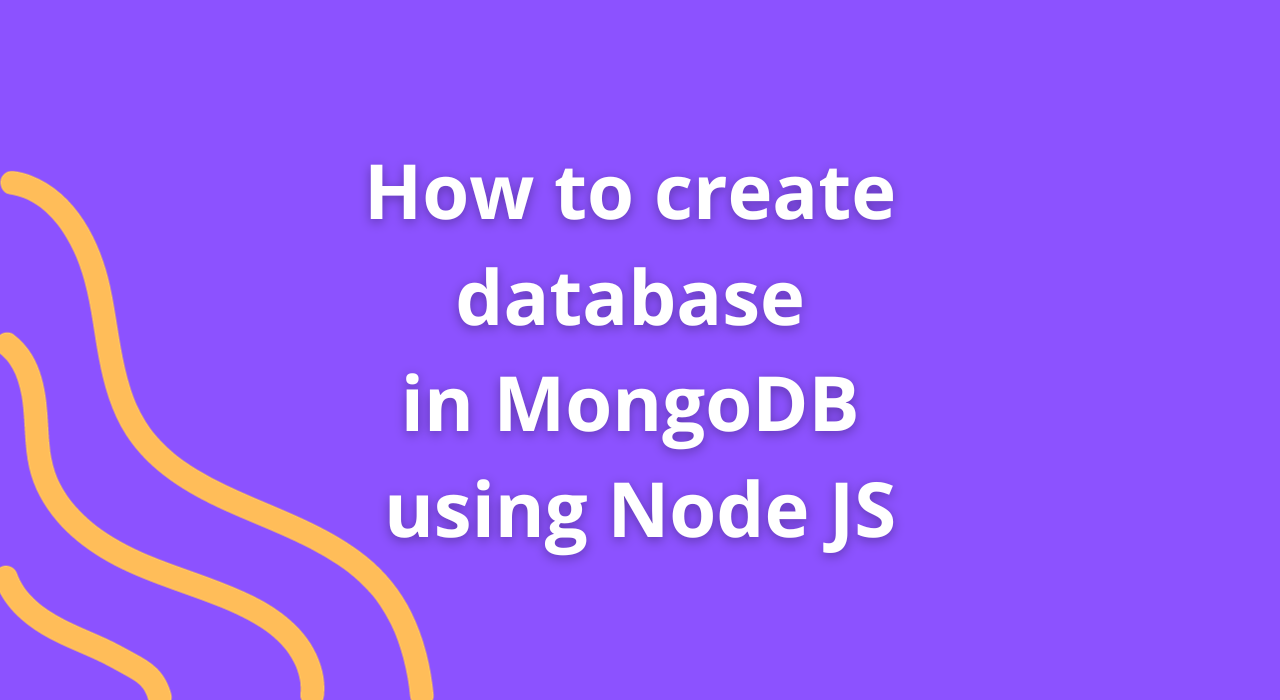
Creating a database in MongoDB using Node.js involves establishing a connection to the MongoDB server and executing commands to create the database. Let’s walk through the steps in a comprehensive guide:
Creating a Database in MongoDB Using Node.js: A Step-by-Step Tutorial
MongoDB, a popular NoSQL database, seamlessly integrates with Node.js, allowing developers to create databases effortlessly. This tutorial will guide you through the process of creating a database in MongoDB using Node.js.
Prerequisites
Ensure you have the following installed:
- Node.js: Installed on your machine
- MongoDB: Installed and running as a service
Setting Up Node.js Project
1. Initialize a Node.js Project
Create a new directory for your project and initialize it with npm:
mkdir mongo-nodejs
cd mongo-nodejs
npm init -y
2. Install MongoDB Driver for Node.js
Install the MongoDB driver to interact with the MongoDB database:
npm install mongodb
Establishing Connection to MongoDB
Create a JavaScript file (e.g., app.js
) and establish a connection to MongoDB:
const { MongoClient } = require('mongodb');
// Connection URL and Database Name
const url = 'mongodb://localhost:27017';
const dbName = 'myDatabase'; // Replace 'myDatabase' with your desired database name
// Create a new MongoClient
const client = new MongoClient(url, { useNewUrlParser: true, useUnifiedTopology: true });
// Connect to the MongoDB server
client.connect((err) => {
if (err) {
console.error('Error occurred while connecting to MongoDB:', err);
return;
}
console.log('Connected successfully to MongoDB server');
// Create a new database
const db = client.db(dbName);
// Perform operations using the db object
// (e.g., create collections, insert documents, etc.)
// Close the connection
client.close();
});
Creating a Database
To create a database in MongoDB using Node.js, perform operations within the connected MongoDB instance:
// Inside the 'client.connect' block
// Create a new database
const db = client.db(dbName);
// You've connected to the database at this point
// If you want to create a collection within the database
db.createCollection('myCollection', (err, res) => {
if (err) {
console.error('Error creating collection:', err);
return;
}
console.log('Collection created successfully');
});
Conclusion
Congratulations! You’ve successfully created a connection to MongoDB using Node.js and created a database within it. You can further expand on this foundation by performing additional operations like inserting documents, querying data, or creating more collections.
MongoDB’s flexibility combined with Node.js allows for seamless database operations and empowers developers to build powerful applications with ease.
Experiment with different MongoDB functionalities within your Node.js applications and explore the vast possibilities of data storage and retrieval offered by this powerful combination.
Happy coding with MongoDB and Node.js! 🌟🚀