How to build a simple REST API in PHP
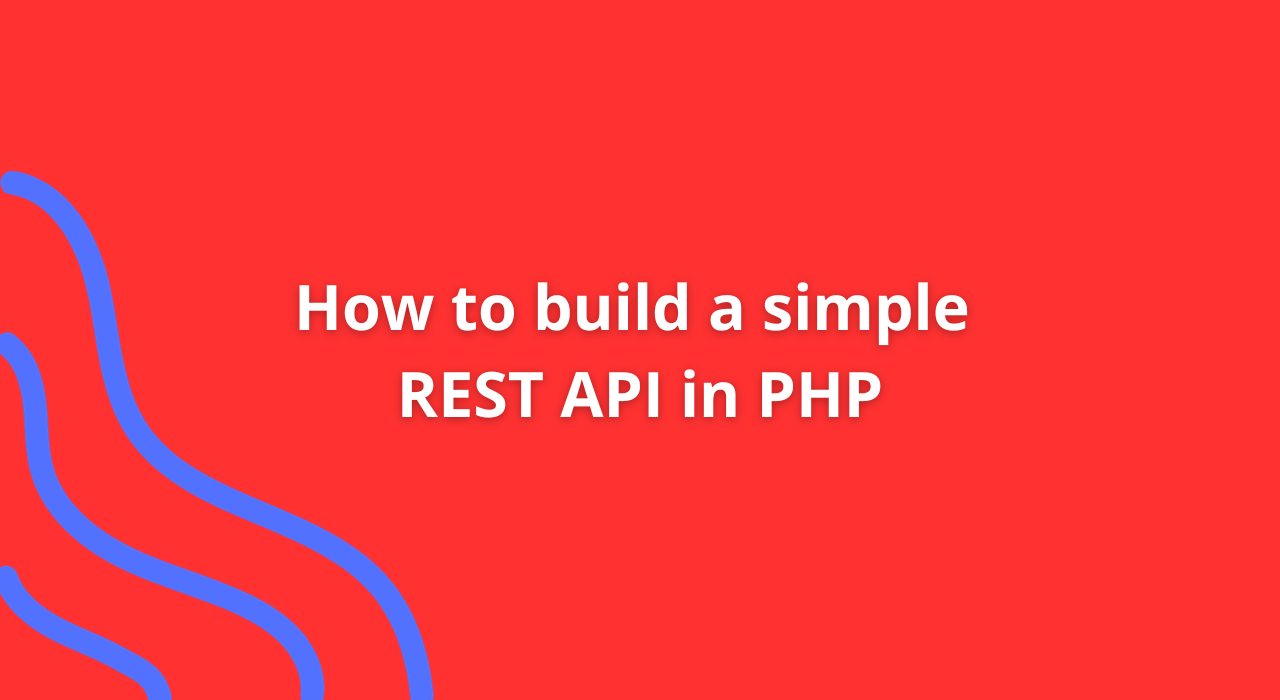
RESTful APIs serve as a backbone for communication between different applications. Creating a basic REST API in PHP is a great way to understand the fundamentals of API development. Let’s walk through the steps to build a simple REST API using PHP.
Setting Up the Project
1. Create Project Directory
Start by creating a new directory for your PHP project.
mkdir simple-rest-api
cd simple-rest-api
2. Project Structure
Create PHP files for your API endpoints:
index.php
: Entry point for routing API requests.users.php
: Handling user-related API endpoints.
Implementing the REST API Endpoints
1. index.php
– Entry Point
This file will handle incoming requests and route them to the appropriate endpoint.
<?php
$requestMethod = $_SERVER['REQUEST_METHOD'];
$requestUri = $_SERVER['REQUEST_URI'];
// Routing requests
switch ($requestUri) {
case '/users':
include 'users.php';
break;
default:
http_response_code(404);
echo json_encode(['error' => 'Endpoint not found']);
break;
}
2. users.php
– User Endpoint
Define the logic for the /users
endpoint to handle GET requests for user data.
<?php
$users = [
['id' => 1, 'name' => 'John'],
['id' => 2, 'name' => 'Alice'],
['id' => 3, 'name' => 'Bob'],
// Add more users as needed
];
if ($_SERVER['REQUEST_METHOD'] === 'GET') {
header('Content-Type: application/json');
echo json_encode($users);
}
Testing the REST API
1. Start PHP Server
Run the PHP built-in server in your project directory:
php -S localhost:8000
2. Access API Endpoint
Navigate to http://localhost:8000/users
in your browser or use tools like Postman to send GET requests to retrieve user data from the API.
Conclusion
Building a simple REST API in PHP involves creating endpoints that handle incoming requests and return appropriate responses. This basic example demonstrates the structure and functionality of a RESTful API using PHP.
Expand upon this foundation by incorporating additional endpoints, implementing CRUD operations, connecting to databases, and adding authentication to create more sophisticated APIs.
Understanding the principles of RESTful APIs and their implementation in PHP lays a solid groundwork for creating powerful web services.
You Might Also Like
- Lorem ipsum dolarorit ametion consectetur
- The Blues Kitchen woks Podcast
- Chasing Dreams in Slow Motion
- If you use this site regularly and would like to help keep the site on the Internet,
- Dolarorit ametion consectetur elit.
- Modern Office Must-Have in 2021
- If you are going to use a passage of Lorem
- Lorem ipsum consectetur elit.