How to auto scroll flatlist in React Native
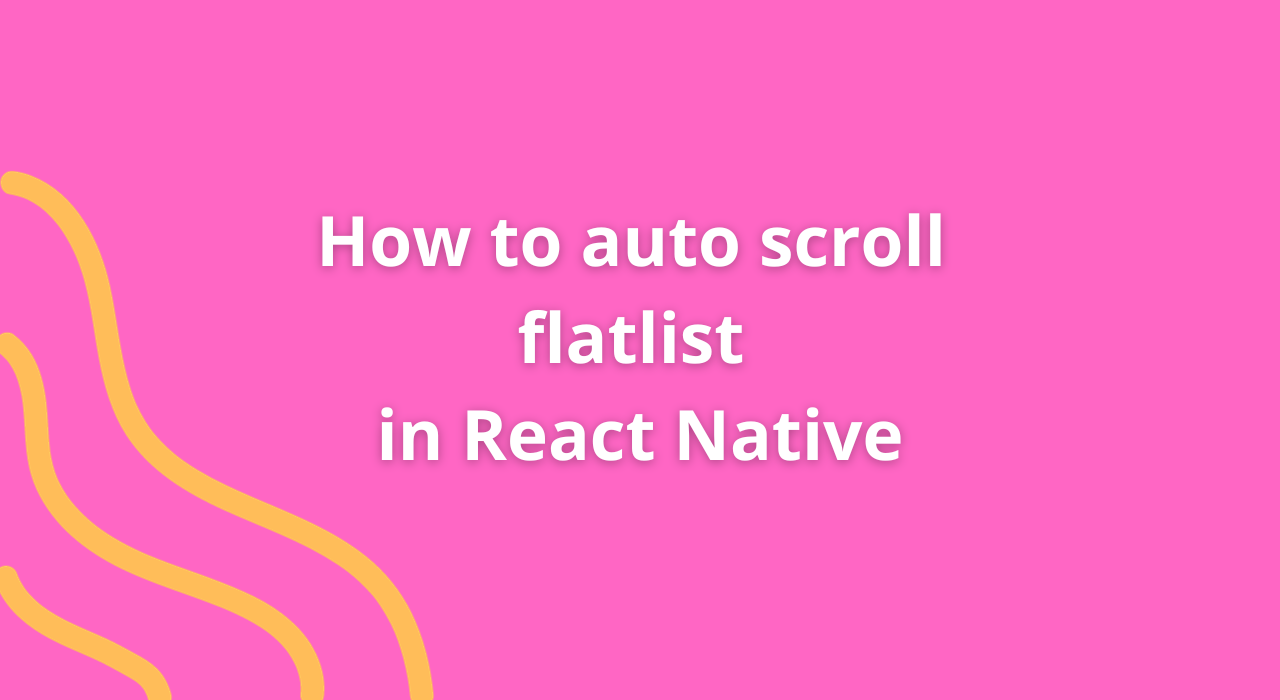
Auto-scrolling a FlatList
in React Native enables automatic movement through a list of content, enhancing user interaction and engagement within mobile applications. Implementing this feature allows for a smoother and more dynamic viewing experience. Let’s explore how to achieve auto-scroll functionality within a FlatList
component in React Native.
Setting Up Auto-Scroll in FlatList
1. FlatList Component Setup
Initialize a FlatList
component within your React Native application:
import React from 'react';
import { FlatList, View, Text } from 'react-native';
const AutoScrollFlatList = () => {
// Data for the FlatList
const data = [
{ id: '1', text: 'Item 1' },
{ id: '2', text: 'Item 2' },
// Add more data as needed
];
// Render item function for FlatList
const renderItem = ({ item }) => (
<View>
<Text>{item.text}</Text>
</View>
);
return (
<FlatList
data={data}
renderItem={renderItem}
keyExtractor={item => item.id}
// Additional FlatList props
/>
);
};
export default AutoScrollFlatList;
2. Implement Auto-Scroll Logic
Use setTimeout
or other methods to achieve auto-scroll behavior by updating the FlatList
‘s scrollToIndex
method:
import React, { useRef, useEffect } from 'react';
import { FlatList, View, Text } from 'react-native';
const AutoScrollFlatList = () => {
// ...
const flatListRef = useRef(null);
useEffect(() => {
const interval = setInterval(() => {
// Calculate the next index to scroll to
// Example: const nextIndex = calculateNextIndex();
// Scroll to the calculated index
flatListRef.current.scrollToIndex({ index: nextIndex, animated: true });
}, 3000); // Adjust the interval duration as needed
return () => clearInterval(interval);
}, []);
return (
<FlatList
ref={flatListRef}
data={data}
renderItem={renderItem}
keyExtractor={item => item.id}
// Additional FlatList props
/>
);
};
export default AutoScrollFlatList;
Conclusion
Implementing auto-scroll functionality within a FlatList
in React Native involves setting up the FlatList
component and incorporating logic to automatically scroll through the list at specified intervals. This feature enhances user engagement by dynamically navigating through content without manual interaction.
By incorporating auto-scrolling capabilities, React Native developers can create more interactive and engaging mobile applications, allowing users to effortlessly browse through content. Integrate these steps to implement auto-scroll functionality within your FlatList
, providing a seamless and captivating user experience in your React Native projects.