How to add Font Awesome icons in Javascript
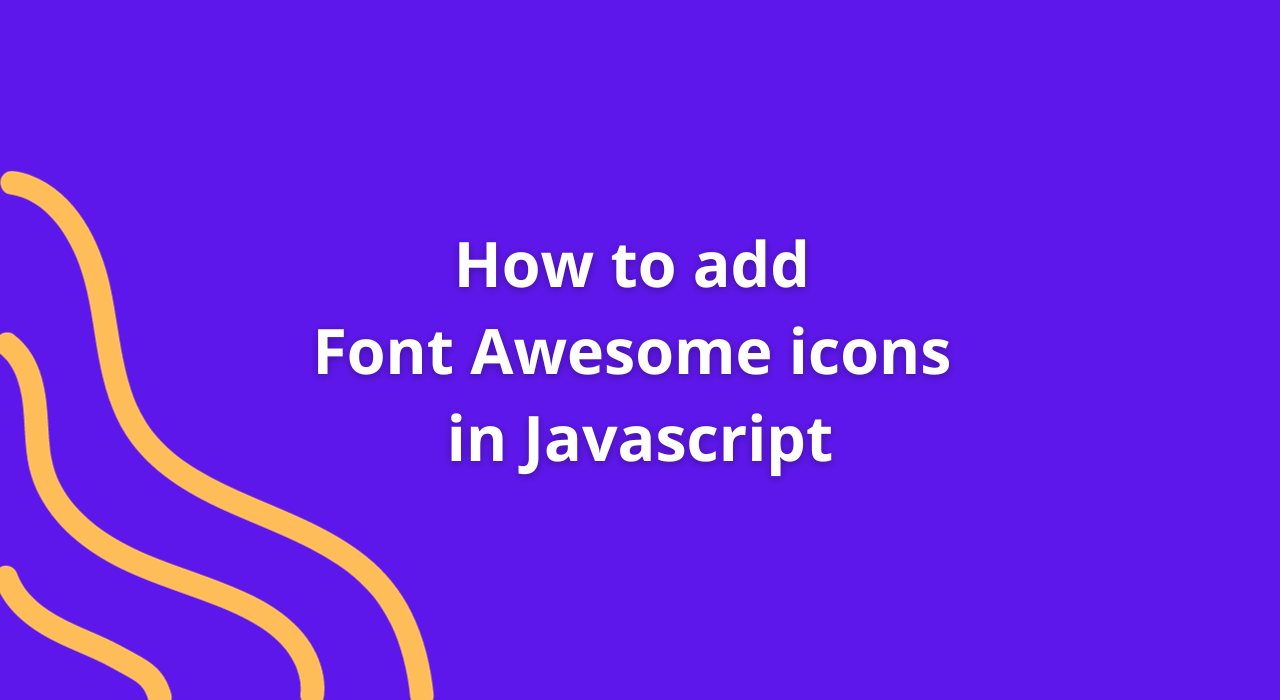
Font Awesome, a popular library for scalable vector icons, provides a convenient way to include icons in web applications. Incorporating Font Awesome icons using JavaScript enables dynamic insertion of icons into HTML elements, enhancing visual elements and user experiences. Let’s explore how to add Font Awesome icons using JavaScript.
Including Font Awesome in HTML
1. Link Font Awesome Library
Start by linking the Font Awesome library in the HTML file’s <head>
section:
<head>
<!-- Other meta tags and styles -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.4/css/all.min.css">
</head>
Adding Font Awesome Icons with JavaScript
1. Using DOM Manipulation
<body>
<div id="icon-container"></div>
<script>
// Create an icon element
const icon = document.createElement('i');
icon.classList.add('fas', 'fa-heart'); // Add Font Awesome classes for the desired icon
// Append the icon to a container element
const iconContainer = document.getElementById('icon-container');
iconContainer.appendChild(icon);
</script>
</body>
createElement()
creates an<i>
element for the icon.classList.add()
adds Font Awesome classes (fas
for solid icons,fa-heart
for the heart icon) to the created element.appendChild()
appends the created icon element to a container (here,<div id="icon-container">
).
2. Using Template Strings
<body>
<div id="icon-container"></div>
<script>
const iconContainer = document.getElementById('icon-container');
iconContainer.innerHTML = `<i class="fas fa-star"></i>`; // Replace with desired Font Awesome icon
</script>
</body>
innerHTML
directly sets the HTML content of the container element to include the desired Font Awesome icon using template strings.
Conclusion
Incorporating Font Awesome icons using JavaScript allows for dynamic insertion and manipulation of icons within web applications. Leveraging Font Awesome’s extensive icon library enhances visual elements and user interfaces, providing scalable vector icons for various purposes.
Happy Coding !